Flask Overview: What kind of framework is Flask?
Table of Contents
- Introduction
- Advantages of the Flask Framework
- Understanding the Core Concepts
- Get started with the Flask Framework
- Working with Flask: Routes, Templates, and Requests
- Using Flask to Create a Simple Web Application
- Extending Flask with Third-Party Add-Ons
- Conclusion
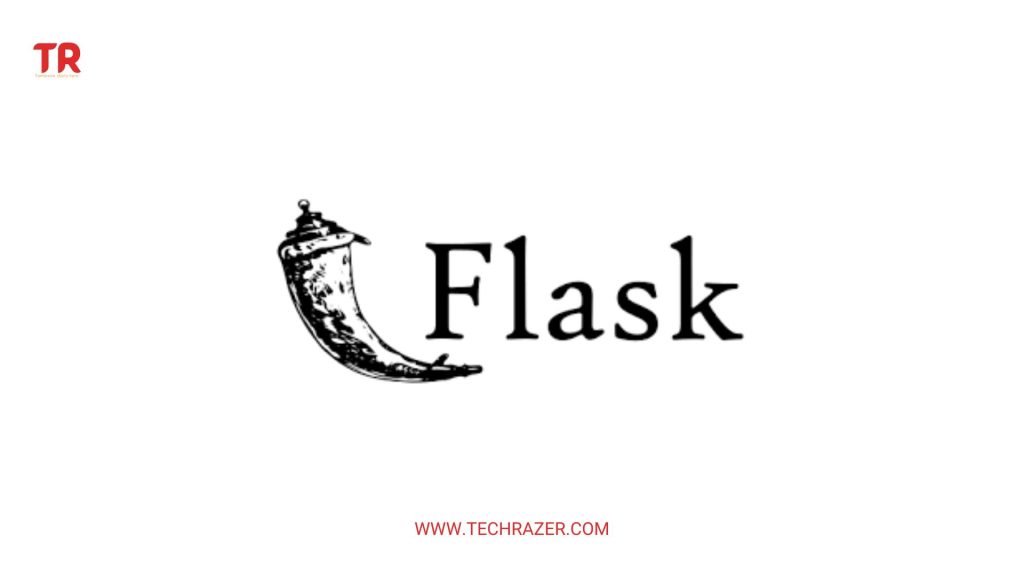
Introduction
Flask is a micro web framework written in Python. Its primary purpose is to help you create web applications quickly and easily. It is based on the Werkzeug WSGI toolkit and Jinja2 template engine. Flask is a lightweight web application framework written in Python. It is designed to make getting started quick and easy, with the ability to scale up to complex applications.
Flask is a web framework built on top of the Python programming language. It was created by Armin Ronacher in 2010 and is maintained by the Pallets Projects. Flask is a microframework that provides the basics for web development without the need for a large, complex framework like Django.
Flask allows developers to easily build web applications by providing the tools needed to create a web application. Flask is often referred to as a ‘microframework’ because it doesn’t require much code to get a basic web application up and running. Flask is also extensible, meaning that developers can add additional features and functionality to their applications.
Flask is a great choice for developers who want to create web applications quickly and easily. It is simple and lightweight, and provides the basic components needed to build web applications. It is also highly extensible, allowing developers to add additional features and functionality as needed.
Flask is used for creating web applications such as websites and blogs, as well as web APIs and services. Flask is often chosen for its simplicity and flexibility, as well as its ability to scale to complex projects. It is also popular for its pluggable architecture, which allows developers to easily customize and extend the framework.
Flask is a great choice for developers who want to create web applications quickly and easily. It is simple and lightweight, and provides the basic components needed to build web applications. It is also highly extensible, allowing developers to add additional features and functionality as needed. Flask is used for creating web applications such as websites and blogs, as well as web APIs and services. Flask is often chosen for its simplicity and flexibility, as well as its ability to scale to complex projects. It is also popular for its pluggable architecture, which allows developers to easily customize and extend the framework.
Flask also supports a variety of databases, including MySQL, SQLite, and PostgreSQL. Flask also supports the popular web template language Jinja2. Flask includes a built-in development server and debugging support, making it easier for developers to quickly and easily build web applications.
Flask is a great choice for developers who want to create web applications quickly and easily. It is simple and lightweight, and provides the basic components needed to build web applications. It is also highly extensible, allowing developers to add additional features and functionality as needed. Flask is used for creating web applications such as websites and blogs, as well as web APIs and services. Flask is often chosen for its simplicity and flexibility, as well as its ability to scale to complex projects. It is also popular for its pluggable architecture, which allows developers to easily customize and extend the framework.
Flask is a great choice for developers who are just getting started with web development, as well as experienced developers looking to create web applications quickly and easily. It is lightweight and extensible, making it easy to customize and extend the framework. Flask is used for creating web applications such as websites and blogs, as well as web APIs and services.
Advantages of the Flask Framework
- Easy to Learn and Use: Flask is very easy to learn and use. It has a minimalistic design which makes it easy to understand and get started with. It also has a lot of documentation and tutorials available online so developers can learn quickly.
- Lightweight: Flask is a lightweight framework which makes it ideal for creating small applications or prototypes. It does not have a lot of dependencies, which makes it easy to deploy and maintain.
- Flexible: Flask is a very flexible framework which allows developers to customize it according to their needs. It is designed to be extensible, so developers can add additional functionality as needed.
- Secure: Flask is a secure framework. It provides a number of built-in protection features, such as input validation, cross-site scripting protection, and secure session management.
- Scalable: Flask is a highly scalable framework. It can handle a large number of requests without any performance issues.
- Fast Development: Flask allows developers to create web applications quickly and easily. It has a built-in development server and debugger, which makes it easy to develop and test applications.
- Cross-Platform: Flask is a cross-platform framework, which means it can be used on any operating system.
Understanding the Core Concepts
The core concepts of the Flask framework are:
- Application Factory
The Application Factory pattern is an important concept in Flask. It is a way of organizing the application and its components. The Application Factory is used to create an instance of the application, as well as all the necessary components such as routes, views, and models. It also helps manage the configuration of the application, as well as the database connections.
- Blueprints
A Blueprint is a way of organizing the application’s components into a modular structure. It is a way of breaking down the application into smaller, more manageable parts. Blueprints allow developers to create reusable application components, such as routes, views, and models.
- Configuration
Configuration is an important part of any web application. Flask allows developers to easily configure the application using a configuration file. This file contains all the necessary settings and options that the application needs to run.
- Templates
Templates are used to create the user interface of the application. Flask uses the Jinja2 template language to create the HTML, CSS, and JavaScript of the application. Templates are used to create the HTML, CSS, and JavaScript that the user sees in the browser.
- URL Routing
URL routing is used to define the URLs that the application will respond to. Routes are used to define the URLs that the application will respond to, and the logic that will be executed when a request is made to that URL.
- Request/Response
The Request/Response cycle is the process that is used when a user makes a request to the application. When a user makes a request, the application receives the request and sends back a response. The response is typically an HTML page or other content that is displayed in the browser.
- Middleware
Middleware is a way of adding functionality to the application without changing the application’s code. It is used to add functionality such as authentication, logging, and caching.
These are the core concepts of the Flask framework.
Get started with the Flask Framework
Step 1: Installing Flask
The first step is to install the Flask framework. This can be done using the Python package manager, pip.
Step 2: Creating the Flask Application
The next step is to create a new Flask application. This can be done by creating a new Python file and importing the Flask module.
Step 3: Defining the Routes
The third step is to define the routes for the application. This can be done using the @app.route() decorator.
Step 4: Defining the Views
The fourth step is to define the views for the application. This can be done by defining functions that return HTML responses.
Step 5: Running the Application
The fifth step is to run the application. This can be done using the flask run command.
Step 6: Testing the Application
The final step is to test the application. This can be done using the built-in development server and debugger.
These are the general steps used to get started with the Flask framework. Of course, there are many other things one must consider when developing a web application, such as security, scalability, and performance. However, these are the basic steps one must follow in order to get started with the Flask framework.
Working with Flask: Routes, Templates, and Requests
This tutorial will cover the basics of working with Flask, including routing, templates, and requests.
Routing
Routing is a key concept when working with Flask. Routing allows a web application to route user requests to the proper web page or action. When a user requests a web page from a server, the server will look for a route that matches the request. If a route is found, the server will then call the associated view or action.
Flask uses a decorator-based syntax to define routes. A decorator is a special type of function that can be attached to a view or action. This allows for easy definition of routes within the application.
Flask uses the @app.route() decorator to define routes. This decorator takes two parameters: the URL path and the view or action to call. For example, the following code defines a route that maps to the index view:
@app.route('/')
def index():
return 'Welcome to the index page!'
Once defined, the index view can be accessed with a web browser by visiting the URL http://localhost:5000/.
Templates
Templates are a key concept in web development. Templates allow developers to separate the presentation layer from the logic layer of their applications. This makes it easier to maintain and update the application as it grows.
Flask uses the Jinja2 template engine for rendering templates. Jinja2 is a powerful template engine that allows developers to easily create dynamic web pages. To render a template, you need to create a template file in the templates folder. For example, the following code creates a template file called index.html:
<html>
<head>
<title>My Home Page</title>
</head>
<body>
<h1>Welcome to my Home Page!</h1>
</body>
</html>
Once the template file is created, you can render it using the render_template() function:
@app.route('/')
def index():
return render_template('index.html')
Requests
The request object is a key concept in web development. The request object is an object that contains information about the current request. This object can be used to access form data, query strings, cookies, and other information about the request.
Flask provides a convenient way to access the request object with the request object. The request object provides access to the form data, query strings, cookies, and other information in the request. For example, the following code can be used to access the query string in the request:
@app.route('/')
def index():
query_string = request.args.get('q')
return 'Query string: ' + query_string
Using Flask to Create a Simple Web Application
Creating a web application using the Flask framework in Python is a relatively simple task. Here are the steps you can follow to create a basic web application using Flask:
- Install Flask: Before you can start building your web application, you’ll need to install Flask. You can do this using pip, the Python package manager, by running the following command:
$ pip install flask
- Create a new Python file: Open your preferred text editor or IDE and create a new Python file. This file will contain the code for your web application.
- Import the Flask module: At the top of your Python file, you’ll need to import the Flask module. You can do this by adding the following line to your file:
from flask import Flask
- Create a Flask app object: Next, you’ll need to create a Flask app object. This object will contain all of the functionality of your web application. You can create a Flask app object by adding the following line to your Python file:
app = Flask(__name__)
- Define a route: A route is a URL pattern that the web application responds to. You can define a route in your Flask app by using the @app.route decorator. For example, to create a route that responds to the root URL (/), you can add the following code to your Python file:
@app.route('/')
def index():
return 'Hello, World!'
The index function is known as a view function, and it returns a string (in this case, ‘Hello, World!’) that will be displayed in the user’s web browser.
- Run the app: To run your web application, you’ll need to use the flask command-line utility. You can start your web application by running the following command in the terminal:
$ flask run
This will start a development server that you can use to test your web application. You can access the web application by visiting http://localhost:5000 in your web browser.
- Add more routes: You can add additional routes to your web application by defining additional view functions and using the @app.route decorator. For example, to create a route that responds to the /about URL, you can add the following code to your Python file:
@app.route('/about')
def about():
return 'This is a simple web application built with Flask.'
- Use templates: Instead of returning hard-coded strings from your view functions, you can use templates to generate dynamic HTML content. Flask uses the Jinja2 template engine, which allows you to embed Python code in your HTML templates.
To use templates, you’ll first need to create a templates directory in the same directory as your Python file. Inside the templates directory, you can create HTML files that contain Jinja2 templates.
For example, you might create a layout.html template that defines the basic layout of your web application, with placeholders for the page title and content:
Extending Flask with Third-Party Add-Ons
One of the great things about Flask is that it is highly extensible, which means you can use third-party add-ons to add additional functionality to your web application. Here are the steps you can follow to extend Flask with third-party add-ons:
- Find an add-on: There are many third-party add-ons available for Flask, including libraries for handling things like authentication, database connectivity, and form validation. You can find a list of popular Flask add-ons on the Flask website (https://flask.palletsprojects.com/en/2.1.x/extensions/) or by searching online.
- Install the add-on: Once you’ve found an add-on that you want to use, you’ll need to install it. You can do this using pip, the Python package manager, by running the following command:
$ pip install
- Import the add-on: After installing the add-on, you’ll need to import it into your Flask application. You can do this by adding an import statement at the top of your Python file.
For example, if you wanted to use the Flask-SQLAlchemy add-on to connect to a database, you would import it like this:
from flask_sqlalchemy import SQLAlchemy
- Initialize the add-on: After importing the add-on, you’ll need to initialize it. This typically involves creating an object and passing it to the Flask app object using the app.config attribute.
For example, to initialize the Flask-SQLAlchemy add-on, you might do something like this:
db = SQLAlchemy(app)
- Use the add-on: Once you’ve initialized the add-on, you can use its functionality in your Flask application. The specific steps for using an add-on will depend on the specific add-on you are using.
For example, if you are using the Flask-SQLAlchemy add-on to connect to a database, you might create a model class to represent a database table, and then use the db.session object to add and query records in the table.
Here’s an example of how you might use Flask-SQLAlchemy to create a simple “todo” list application:
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///todos.db'
db = SQLAlchemy(app)
class Todo(db.Model):
id = db.Column(db.Integer, primary_key=True)
text = db.Column(db.String(200))
complete = db.Column(db.Boolean)
@app.route('/')
def index():
todos = Todo.query.all()
return render_template('index.html', todos=todos)
@app.route('/add', methods=['POST'])
def add_todo():
todo = Todo(text=request.form['todo_text'], complete=False)
db.session.add(todo)
db.session.commit()
return redirect('/')
@
Conclusion
Flask is a powerful and popular Python microframework used for web development. Flask enables developers to quickly create web applications with minimal effort. This tutorial covered the basics of working with Flask, including routing, templates, and requests. Routing allows a web application to route user requests to the proper web page or action. Templates allow developers to separate the presentation layer from the logic layer of their applications. The request object is an object that contains information about the current request. With these tools, developers can quickly create dynamic web applications using Flask.