Django Framework: A Beginner’s Guide to Building Web Apps
Table of Contents
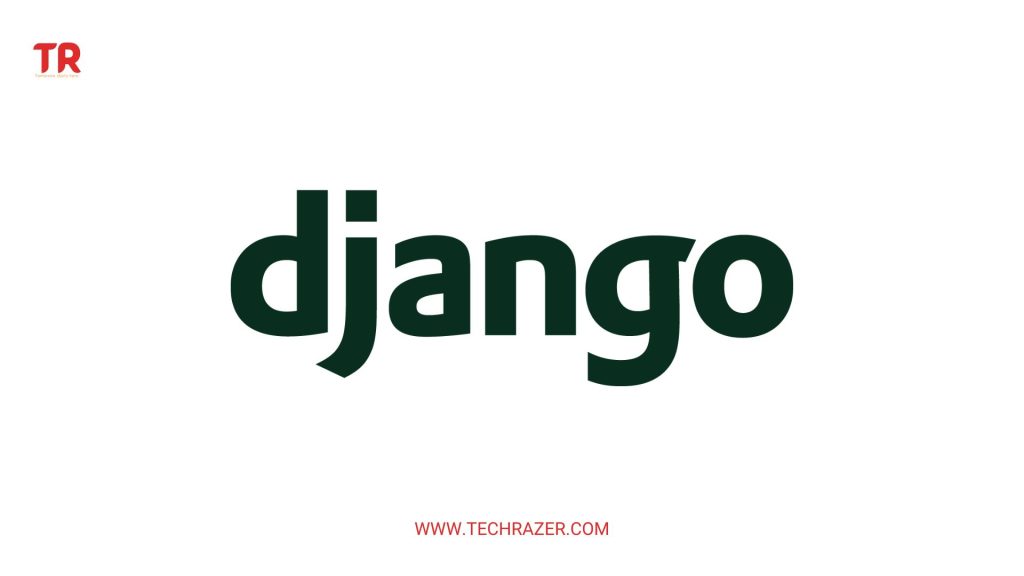
Introduction
Django is an open-source, Python-based web framework that enables developers to quickly create and deploy high-quality, secure, and maintainable web applications. It is a widely used web framework for building efficient, scalable web applications. It has a large and vibrant community of users and developers.
Django is a Model-View-Template framework, which is an architecture pattern to separate the data, view and user interface. It is based on the concept of “Don’t repeat yourself” (DRY), which helps developers to reduce the amount of code they need to write. It is highly extensible and customizable, and it allows developers to create complex web applications using minimal code.
Django provides a wide range of features that make it a great choice for web development. It has an object-relational mapper (ORM) which helps developers to interact with databases without writing SQL queries. It also provides an authentication system which allows developers to create and manage user accounts, as well as permission control. Other features include an easy-to-use URL routing mechanism, a powerful template engine, and a powerful admin interface.
Django is a highly secure web framework. It has an authentication system which allows developers to securely manage user accounts and permissions. It also has a secure and powerful ORM which helps developers to protect their data from malicious attacks. Additionally, Django provides an extensive set of tools for debugging and performance optimization.
Django is easy to learn and use. It has an extensive library of packages and modules which developers can use to quickly create and deploy web applications. It also has a comprehensive official documentation which helps developers to easily understand the framework and its features. Additionally, there are many tutorials and books available which can help developers to learn and understand the framework.
Setting up Django Framework
Requirements for running Django
- Python
- Virtual Environment
- Django
- Database (MySQL, PostgreSQL, SQLite etc.)
- Web Server (Apache, Nginx etc.)
Steps for setting up Django
- Install Python and add it to your system path: Before starting Django installation, make sure you have installed Python on your system and it is available in the system path.
- Install Django: Once Python is installed, you can install Django using the command prompt. To install Django, run the following command:
$ pip install Django
- Configure a Database: After installing Django, the next step is to configure a database to use with it. If you are using a relational database such as MySQL, PostgreSQL, or SQLite, you will need to install a database adapter.
- Create a Project: Once the database is configured, you can create a Django project using the command line. To create a project, run the following command:
$ django-admin start:project myproject
- Set up the Settings: Next, you will need to set up the settings for the project. The settings file contains information such as the database configuration, installed applications, and the URL configuration. The settings file is located in the project’s root directory.
- Create an App: After setting up the settings, you will need to create an application within the project. To create an app, run the following command:
$ python manage.py start:app myapp
- Configure the URLs: Next, you will need to configure the URLs for the project. The URL configuration is defined in the urls.py file in the project’s root directory.
- Set up the Views: Once the URLs are configured, you will need to set up the views for the project. The views are responsible for rendering the content to the user. To create a view, create a file called views.py in the project’s root directory and add the following code:
def index(request):
return render(request, 'index.html')
- Set up the Templates: The last step is to create the templates for the project. The templates are responsible for displaying the content to the user. Create a folder called templates in the project’s root directory and add a file called index.html with the following code:
<html>
<head>
<title>My Project</title>
</head>
<body>
<h1>Welcome to My Project!</h1>
</body>
</html>
- Run the Server: Once all the above steps are completed, you can run the server and access the project. To run the server, run the following command:
$ python manage.py run:server
Django Features
Here, we explain the steps of the Django features.
- Model – The model layer is the foundation of a Django project. It is responsible for defining the data structure for the application and managing the data. This includes creating models, making migrations, and defining relationships between models.
- View – The view layer is responsible for handling requests and returning responses. It defines the logic of the application and is responsible for rendering templates. Views are typically written as functions or classes and can use the Django template language to generate responses.
- Template – The template layer is responsible for generating HTML or other types of output. It uses the Django template language to create templates that can be rendered to HTML or other formats. Templates can be used to generate dynamic content and can be extended with custom tags and filters.
- URLconf – The URLconf layer is responsible for mapping URLs to views. It defines the URL patterns for the project and can be used to generate unique URLs for each view. URLs can be used to generate dynamic content and can also be used to validate user input.
- Authentication – Authentication is responsible for handling user authentication and authorization. It provides methods for authenticating users, managing user sessions, and enforcing access control. Authentication can be used to secure data and can be integrated with third-party authentication services.
- Forms – Forms are responsible for capturing user input and validating it. They can be used to create HTML forms, process submitted data, and validate user input. Forms can also be used to generate dynamic forms based on user input.
- Middleware – Middleware is responsible for processing requests and responses. It can be used to modify the request and response objects, handle exceptions, and perform other processing tasks. Middleware can be used to perform authentication and authorization tasks or to add custom functionality to an application.
- Admin – The admin layer is responsible for managing the application. It provides an interface for creating, updating, and deleting models and allows users to manage the application data. The admin layer also provides tools for customizing the application and for managing users, groups, and permissions.
- Internationalization – Internationalization is responsible for handling multiple languages. It provides tools for creating translations of strings and templates and for managing different language versions of an application. It can also be used to localize content and to provide language-specific features.
- Caching – Caching is responsible for improving the performance of an application. It can be used to store data in memory or on disk, to reduce the number of database queries, and to reduce the time spent generating dynamic content. Caching can be used to improve the response time of an application and to reduce server load.
These are the steps of the Django features. By following these steps, developers can quickly create secure and maintainable websites. Django provides a full-stack solution for web development and can be used to create powerful web applications.
Benefits of Django Framework
- Quick Development:
Django is a high-level Python web framework that enables rapid development of applications. This makes it possible to create complex, database-driven websites in a short period of time. With Django?s component-based architecture and its powerful ORM, developers can quickly build web applications with minimal effort. Furthermore, the framework provides a number of out-of-the-box features that can be used to speed up the development process. - Secure:
Django is designed with security in mind. It provides a secure environment for web applications with built-in protection against common security threats such as cross-site scripting (XSS), cross-site request forgery (CSRF), SQL injection, and clickjacking. It also offers user authentication and authorization tools to help developers secure their applications. - Scalable:
Django is built to scale. It can handle millions of requests per second, making it an ideal choice for applications that need to process large amounts of data. Additionally, Django?s modular architecture allows developers to easily add new features and customize existing ones. - Flexible:
Django is a highly flexible framework. It gives developers the freedom to customize existing components and create new ones. This means that developers can tailor the framework to their specific needs and create applications that are unique and powerful. - Documentation:
Django has extensive documentation that is easy to understand and follow. This makes it easy for developers to get up and running quickly and start building applications. Additionally, the framework has a vibrant community of developers who are always willing to help and answer questions. - Open Source:
Django is an open source framework, which means it is free to use and modify. This makes it an attractive option for developers who are looking to save money and time. Additionally, developers can contribute to the framework, which helps to keep it up to date and secure. - Versatile:
Django can be used to create a variety of applications, from basic websites to complex web applications. It also supports popular databases, making it easy to integrate with existing systems. Furthermore, the framework can be used to create mobile applications and APIs.
Conclusion
In conclusion, Django is a powerful, efficient, and secure web framework which makes it a great choice for web development. It is easy to learn and use, and it has an extensive library of packages and modules which make it highly extensible and customizable. It provides a secure authentication system which helps developers to protect their data from malicious attacks. Additionally, it provides an easy-to-use URL routing mechanism, a powerful template engine, and an admin interface. All in all, Django is a great choice for web development.