A beginner’s guide to building a mobile app with React Native
Table of Contents
- Introduction to React Native
- What is React Native?
- How Does React Native Work?
- Benefits of Using React Native
- Examples of Successful Apps Built with React Native
- Setting up a React Native development environment
- Basic concepts of React Native
- Components and JSX syntax used in React Native
- Building a simple React Native app
- Conclusion
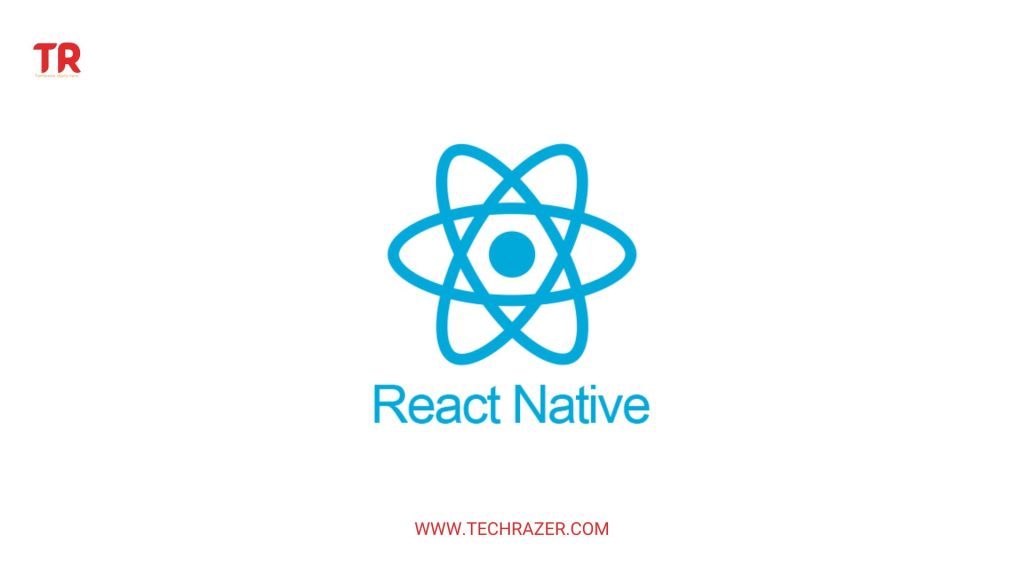
Introduction to React Native
React Native is a popular framework for building mobile applications that run on both Android and iOS devices. Developed by Facebook, it allows developers to use the same codebase for building native apps for both platforms, saving time and resources.
React Native works by using native components for rendering instead of using a web view, which is what most hybrid mobile app frameworks do. This means that the app will have a similar look and feel to a native app, and will also be able to access the native device features such as the camera and push notifications.
To use React Native, developers write their code in JavaScript, using the React framework to build the user interface and to manage the state of the app. The React Native runtime then takes this code and translates it into native platform-specific components, which are rendered on the device.
One of the key benefits of using React Native is that it allows developers to build mobile apps that perform similarly to native apps, while still being able to use the same codebase across multiple platforms. This means that developers can build an app that works on both iOS and Android using the same codebase, rather than having to maintain separate codebases for each platform.
In this blog, we will delve into the basics of React Native, its features, and how it compares to other mobile development frameworks. We will also look at some examples of successful apps built with React Native and discuss its future prospects.
What is React Native?
React Native is an open-source framework that uses the popular JavaScript library React to build native mobile applications. It allows developers to build native apps that feel and perform like native apps, using the same design principles and user experience as native apps.
React Native was first introduced in 2015 and has since become one of the most popular frameworks for building mobile apps. It has a large and active community of developers, and there are many resources available for learning and using the framework.
How Does React Native Work?
React Native uses a hybrid approach to mobile app development. It combines the benefits of native app development with the ease of use and flexibility of web development.
On the one hand, React Native uses native components and APIs to access device features and capabilities, such as the camera, GPS, and push notifications. This allows developers to build apps that feel and perform like native apps.
On the other hand, React Native uses a declarative programming model and a virtual DOM (Document Object Model) to render the user interface. This means that developers can use the same codebase to build apps for both Android and iOS, and the framework will automatically adapt the code to the specific platform.
Benefits of Using React Native
There are several benefits to using React Native for mobile app development:
- Cross-platform compatibility: React Native allows you to build applications that can run on both iOS and Android platforms, saving you time and resources by not having to build separate versions of your app for each platform.
- Improved performance: React Native applications have the potential to perform as well as natively written applications, thanks to its use of native components and its ability to access native APIs.
- Reusable code: Because React Native allows you to build applications using the same codebase for both iOS and Android, you can reuse code between the two platforms, reducing the amount of time and effort required to build and maintain your application.
- Strong developer community: React Native has a large and active developer community, which means there are many resources available for learning and getting help with your projects.
- Ease of use: React Native uses a declarative style of programming, which makes it easy for developers to reason about the state of their application and how it should behave. It also includes a powerful developer toolset that makes it easy to debug and optimize your application.
- Hot reloading: React Native includes a feature called “hot reloading,” which allows you to see changes you make to your code immediately in the app, without having to manually rebuild and deploy the app. This can greatly speed up the development process.
Examples of Successful Apps Built with React Native
There are many successful apps that have been built with React Native. Some examples include:
- Facebook: The Facebook app was one of the first apps to be built with React Native. The company has continued to use the framework to build new features and improve the performance of the app.
- Instagram: Instagram has also used React Native to build several features for its app, including the ability to share multiple photos and videos in a single post.
- Airbnb: Airbnb used React Native to build its mobile app, which allows users to find and book vacation rentals around the world.
- Skype: Microsoft used React Native to build a new version of Skype for iOS and Android, which has improved performance and a modern user interface.
Setting up a React Native development environment
To get started with React Native, you’ll need to have the following tools installed on your development machine:
- Node.js: React Native is built with Node.js, so you’ll need to have it installed on your system. You can download the latest version of Node.js from the official website (https://nodejs.org/) or through a package manager such as Homebrew (on macOS) or Chocolatey (on Windows).
- npm: npm is the package manager for Node.js, and it comes bundled with Node.js. You can use npm to install React Native and other dependencies for your project.
- React Native CLI: The React Native CLI (Command Line Interface) is a tool that helps you set up and run a React Native project. You can install the React Native CLI by running the following command:
$ npm install -g react-native-cli
- A code editor: You’ll need a code editor to write your React Native code. Some popular options include Visual Studio Code, Sublime Text, and WebStorm.
- Xcode (for iOS development): If you want to develop React Native apps for iOS, you’ll need to have Xcode installed on your Mac. Xcode is available for free on the Mac App Store.
- Android Studio (for Android development): If you want to develop React Native apps for Android, you’ll need to have Android Studio installed on your machine. Android Studio is available for free on the official website (https://developer.android.com/studio).
Once you have these tools installed, you can create a new React Native project by running the following command:
$ react-native init MyApp
This will create a new directory called “MyApp” with the basic structure for a React Native app. You can then navigate to the directory and start the development server by running the following command:
$ cd MyApp
$ react-native run-ios
This will start the development server and open the app in an iOS simulator (if you’re on a Mac and have Xcode installed). You can also run the app on an Android emulator by running the following command:
$ react-native run-android
I hope this helps! Let me know if you have any questions.
Basic concepts of React Native
React is a JavaScript library for building user interfaces. It was developed by Facebook, and is often used for building mobile applications and single-page applications.
React allows developers to create reusable UI components. It uses a virtual DOM (Document Object Model) to optimize updates to the actual DOM, making it faster and more efficient than other JavaScript libraries or frameworks that manipulate the DOM directly.
React follows a declarative programming model, which means that the developer specifies what they want the UI to look like, and React takes care of updating the DOM to match the desired state. This makes it easy to reason about and debug applications built with React.
React Native is a framework that allows you to use React to build native mobile applications for Android and iOS. It uses the same principles as React, but instead of rendering to the DOM, it renders native views for the specific platform. This allows you to build native mobile applications that have a native look and feel, while still using the power and flexibility of React.
If you’re new to React, it’s recommended to start by learning the basics of the React library before diving into React Native. There are many resources available for learning React, including the official React documentation and various online tutorials and courses.
Components and JSX syntax used in React Native
One of the key features of React Native is JSX, a syntax extension for JavaScript that allows you to write declarative UI components using a syntax that looks similar to HTML. Here is an example of a simple JSX component:
import React from 'react';
import { View, Text } from 'react-native';
const MyComponent = () => (
<View>
<Text>Hello, world!</Text>
</View>
);
In this example, the View
and Text
components are part of the React Native library and are used to build the UI of the component. The View
component is equivalent to a div
element in HTML, and the Text
component is used to display text on the screen.
JSX allows you to define the structure and content of your UI components using a declarative syntax, making it easy to create complex layouts and build reusable UI components. You can also use JavaScript expressions and functions within JSX by enclosing them in curly braces, which allows you to dynamically render content based on data or state.
Here is an example of a JSX component that uses a JavaScript expression to render dynamic content:
import React from 'react';
import { View, Text } from 'react-native';
const MyComponent = ({ name }) => (
<View>
<Text>Hello, {name}!</Text>
</View>
);
In this example, the name
prop is passed to the component as an argument and is used to render dynamic content within the Text
component.
In addition to JSX, React Native also includes a number of other components and APIs for building native mobile applications. Some of the key components and features include:
StyleSheet
: A way to style your components using a declarative, flexible API that is similar to CSS.LayoutAnimation
: A way to animate the layout of your components using simple, declarative APIs.Animated
: A set of APIs for animating components using a powerful, declarative animation library.Touchable
: A set of components that provide touchable UI elements, such as buttons and links.Modal
: A way to present content on top of the current screen using modal windows.
React Native also provides a rich set of APIs for working with native platform features, such as accessing the camera, storing data, and interacting with the device’s filesystem.
Component lifecycle and state management
In a React application, a component’s lifecycle refers to the various stages a component goes through from its creation to its destruction. These stages are called lifecycle methods, and they provide the opportunity for a developer to execute code at specific points in a component’s lifecycle.
There are three main phases in a React component’s lifecycle:
- Mounting: This is the process of initializing a new component and rendering it to the DOM. During this phase, the following lifecycle methods are called:
constructor()
: This method is called before the component is mounted. It is typically used to set up the initial state of the component and bind event handlers.render()
: This method is called to render the JSX for the component to the DOM.componentDidMount()
: This method is called after the component has been rendered to the DOM. It is typically used to perform tasks that require the DOM to be fully rendered, such as making AJAX requests or setting up timers.
- Updating: This is the process of updating a component with new props or state. During this phase, the following lifecycle methods are called:
shouldComponentUpdate()
: This method is called before the component is updated. It allows the developer to determine whether the component should re-render or not based on the new props or state.render()
: This method is called to render the JSX for the updated component to the DOM.componentDidUpdate()
: This method is called after the component has been updated and re-rendered to the DOM. It is typically used to perform tasks that require the DOM to be fully rendered, such as making AJAX requests or setting up timers.
- Unmounting: This is the process of destroying a component and removing it from the DOM. During this phase, the following lifecycle method is called:
componentWillUnmount()
: This method is called before the component is unmounted and destroyed. It is typically used to perform tasks such as canceling timers or cleaning up event handlers.
State management refers to the process of managing and updating the data (state) of a component. In React, a component’s state is a JavaScript object that holds the data specific to that component. A component’s state can be updated using setState()
, which triggers a re-render of the component. It is important to manage a component’s state properly, as it determines how the component will behave and render.
There are several approaches to state management in a React application, including using the component’s state, using context, and using Redux. It is important to choose the right approach based on the needs of the application.
Building a simple React Native app
Here is a basic walkthrough of how to create a React Native app with a few screens and navigation between them:
- First, make sure you have React Native installed on your development machine. If you don’t have it installed, you can follow the instructions at https://reactnative.dev/docs/environment-setup to set it up.
- Once you have React Native installed, create a new project by running the following command:
$ react-native init MyApp
This will create a new directory called “MyApp” with the basic files and structure needed for a React Native app.
- Next, navigate to the root directory of the project and start the development server by running the following command:
$ cd MyApp
$ react-native start
- In a separate terminal window, run the following command to launch the app in an emulator or on a physical device:
$ react-native run-android
- Now that the basic setup is complete, you can start building your app. First, let’s create a couple of screens. To do this, create a new directory called “screens” at the root of your project, and then create a new file called “HomeScreen.js” inside the “screens” directory.
- In the “HomeScreen.js” file, create a basic React component that displays a message on the screen:
import React from 'react';
import { View, Text } from 'react-native';
const HomeScreen = () => {
return (
<View>
<Text>Welcome to the Home Screen!</Text>
</View>
);
};
export default HomeScreen;
- Create a second screen by creating a new file called “AboutScreen.js” in the “screens” directory and adding the following code:
import React from 'react';
import { View, Text } from 'react-native';
const AboutScreen = () => {
return (
<View>
<Text>Welcome to the About Screen!</Text>
</View>
);
};
export default AboutScreen;
- Next, you’ll need to set up navigation between the two screens. To do this, you can use a library like React Navigation. To install React Navigation, run the following command:
$ npm install @react-navigation/native
- Once React Navigation is installed, you can set up your navigation stack by modifying the root component of your app. Open the “App.js” file and import the
createStackNavigator
function from React Navigation:
import { createStackNavigator } from '@react-navigation/stack';
- Then, create a stack navigator by calling the
createStackNavigator
function and passing in an object that defines the screens in your app:
const Stack = createStackNavigator();
const App = () => {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="About" component={AboutScreen} />
</Stack.Navigator>
</NavigationContainer>
);
};
- Finally, We have created a simple react native navigation app successfully.
Explanation of how to style components and use layout elements
In React Native, you can style components using a style prop, which takes an object containing the styles for the component. The styles are written in JavaScript, and you can use the same style properties as in CSS, with some differences in the property names (for example, “background-color” becomes “backgroundColor”).
Here is an example of how to style a component in React Native:
import React from 'react';
import { View, Text } from 'react-native';
function MyComponent() {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello World</Text>
</View>
);
}
const styles = {
container: {
backgroundColor: '#fafafa',
padding: 20,
},
text: {
fontSize: 20,
color: '#333',
},
};
export default MyComponent;
You can also use layout elements such as View
and Text
to define the structure and content of your app.
View
is a container element that can be used to group and style other elements.Text
is used to display text content.
Here is an example of how to use View
and Text
to create a simple layout:
import React from 'react';
import { View, Text } from 'react-native';
function MyApp() {
return (
<View style={styles.container}>
<Text style={styles.header}>My App</Text>
<View style={styles.content}>
<Text>Welcome to my app!</Text>
</View>
</View>
);
}
const styles = {
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
},
header: {
fontSize: 20,
fontWeight: 'bold',
marginBottom: 20,
},
content: {
width: '80%',
},
};
export default MyApp;
In this example, the container
View
has a flex
style set to 1, which means it will take up the entire available space. The header
Text
and content
View
are centered within the container
using the alignItems
and justifyContent
styles.
There are many other layout elements and style properties available in React Native, and you can learn more about them in the React Native documentation.
Introduction to using third-party libraries and components
React Native allows you to use third-party libraries and components to add functionality and features to your app. There are many libraries and components available on npm (Node Package Manager) that you can use in your React Native app.
To use a third-party library or component in your React Native app, you will need to install it using npm. For example, to install the react-native-elements
library, you can run the following command in your terminal:
$ npm install react-native-elements
Once you have installed the library, you can import it into your code and use it like any other component. For example:
import React from 'react';
import { View } from 'react-native';
import { Button } from 'react-native-elements';
function MyApp() {
return (
<View>
<Button title="Click me" />
</View>
);
}
export default MyApp;
You can also use third-party components from popular UI libraries such as react-native-paper
and native-base
. These libraries provide a wide range of customizable components that you can use to build the user interface of your app.
It is important to note that you should be cautious when using third-party libraries and components, as they may introduce security vulnerabilities or performance issues to your app. Make sure to research and evaluate the libraries and components you use before integrating them into your app.
Conclusion
Overall, React Native is a powerful and popular framework for building cross-platform mobile applications. It offers a number of benefits, including fast and responsive user interfaces, the ability to use existing knowledge of JavaScript and the React library, and a large and active community of developers. If you are considering building a mobile application, React Native is definitely worth considering.