What is jQuery is used for?
Table of Contents
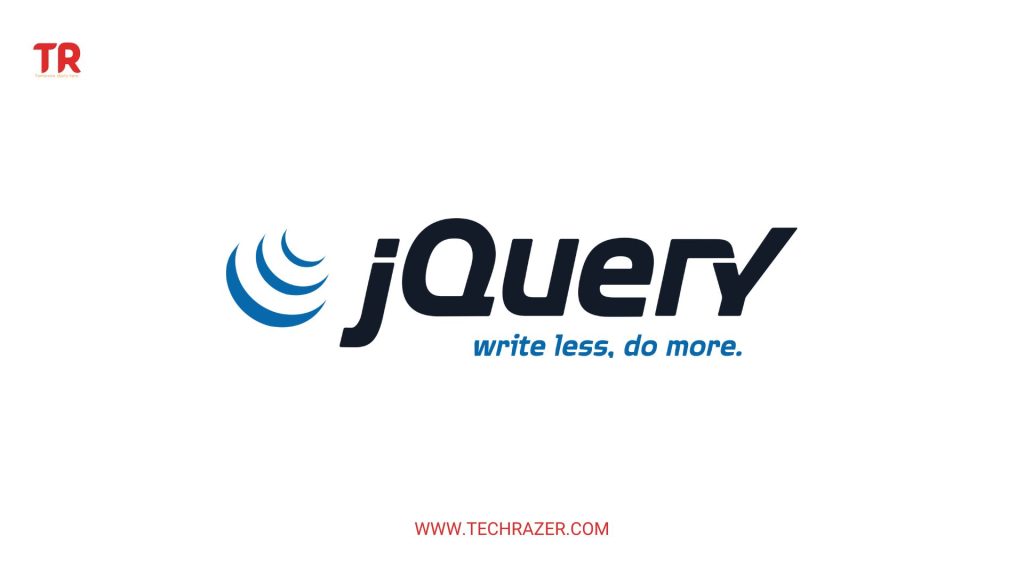
Introduction
jQuery is a fast, small, and feature-rich JavaScript library that makes HTML document traversal and manipulation, event handling, and animation much simpler with an easy-to-use API that works across a multitude of browsers. With a combination of versatility and extensibility, jQuery has changed the way that millions of people write JavaScript.
It was created in 2006 by John Resig and released under the MIT license. It has since become one of the most popular JavaScript libraries used on the Web, with installation on more than 65% of the top 10 million highest-traffic sites on the Web.
One of the most appealing features of jQuery is its simplicity. The library is designed to make it easy for developers to use JavaScript to manipulate the DOM (Document Object Model) and add interactivity to websites. Instead of having to write long lines of complex code, developers can use a few lines of simple, easy-to-understand code to achieve the same result.
For example, to select all the paragraphs in a page and hide them, you could use the following code:
$("p").hide();
To select all the links on a page and add a click event to them, you could use the following code:
$("a").click(function() {
// code to be executed when a link is clicked
});
It also makes it easy to manipulate the DOM. For example, you can use the .html()
function to get or set the HTML content of an element. You can use the .css()
function to get or set the CSS properties of an element. And you can use the .attr()
function to get or set the attributes of an element.
$("#myDiv").html("<p>Hello World!</p>");
$("#myDiv").css("color", "red");
$("#myLink").attr("href", "http://www.example.com");
jQuery also has a rich set of animation effects that you can use to add visual appeal to your website. For example, you can use the .fadeIn()
and .fadeOut()
functions to fade an element in or out. You can use the .slideUp()
and .slideDown()
functions to slide an element up or down. And you can use the .animate()
function to create custom animations.
$("#myDiv").fadeIn();
$("#myDiv").slideUp();
$("#myDiv").animate({left: '250px'});
jQuery also has a large community of developers who have created hundreds of plugins that extend the functionality of the library. These plugins allow you to add all sorts of functionality to your website, from image sliders and carousels to form validation and data tables.
What is jQuery Used For?
jQuery is used for a variety of tasks, including:
- DOM manipulation: One of the main purposes of jQuery is to make it easier to manipulate the DOM of a webpage. This includes tasks such as adding, removing, or modifying elements on the page, as well as changing their style or layout.
- Event handling: jQuery makes it easy to handle events such as clicks, hover events, and form submissions. It provides a simple syntax for attaching event handlers to elements, as well as for triggering events programmatically.
- Animations: jQuery includes a number of methods for creating animations, such as fading, sliding, and rotating elements on the page. These animations can be used to add visual interest to a webpage or to create transitions between different states of an application.
- AJAX: jQuery makes it easy to send and receive data asynchronously from a server, using technologies such as XMLHttpRequest and JSON. This allows developers to create web applications that can update their content without requiring a full page reload.
- Cross-browser compatibility: jQuery is designed to work across a wide range of browsers, including Internet Explorer, Firefox, Chrome, Safari, and others. This makes it easier for developers to create applications that work consistently across different environments.
Why Use jQuery?
There are a number of reasons why jQuery has become so popular among developers:
- Simplicity: jQuery provides a simple and intuitive syntax that makes it easy to select and manipulate elements on a webpage. This makes it easier for developers to get up to speed with the library and to create applications quickly.
- Cross-browser compatibility: As mentioned above, jQuery is designed to work across a wide range of browsers, which means that developers don’t have to worry about writing separate code for each browser. This can save a lot of time and effort, particularly when working on large projects.
- Large community: jQuery has a large and active community of developers who contribute to the library, as well as create and share plugins and other resources. This means that developers can often find solutions to common problems, or add new functionality to their applications, without having to write everything from scratch.
- Ecosystem of plugins: In addition to the core library, there are thousands of plugins available for jQuery that add new functionality or enhance existing features. These plugins can be easily integrated into an application, which means that developers can add new functionality without having to write a lot of code.
jQuery also allows developers to create plugins on top of the JavaScript library. These plugins are used to create powerful dynamic web applications that can be used on any website.
jQuery is used on a variety of web projects, including websites, web applications, and mobile apps. It is used to add interactivity to websites, such as menus, carousels, sliders, forms, and modal windows. It is also used to create dynamic user interfaces, such as tabs, accordions, and autocomplete fields.
jQuery also makes it easier to create web applications by providing a unified API for manipulating the DOM, making AJAX requests, and handling events. This makes it easier for developers to write cross-browser code.
In addition, jQuery makes it easier to create mobile apps by providing tools for creating mobile-friendly user interfaces. jQuery Mobile is a framework for creating mobile apps that are designed to work on all major mobile platforms.
Conclusion
Overall, jQuery is a powerful and versatile JavaScript library that makes it easier to create rich and interactive web applications. It simplifies tasks that would be difficult or complex with plain JavaScript. It provides a unified API for manipulating the DOM, making AJAX requests, and handling events. It also provides tools for creating mobile-friendly user interfaces. With its versatile and extensible nature, jQuery has become one of the most popular JavaScript libraries in the world, used on millions of websites and web applications.