What is ExpressJS used for?
Table of Contents
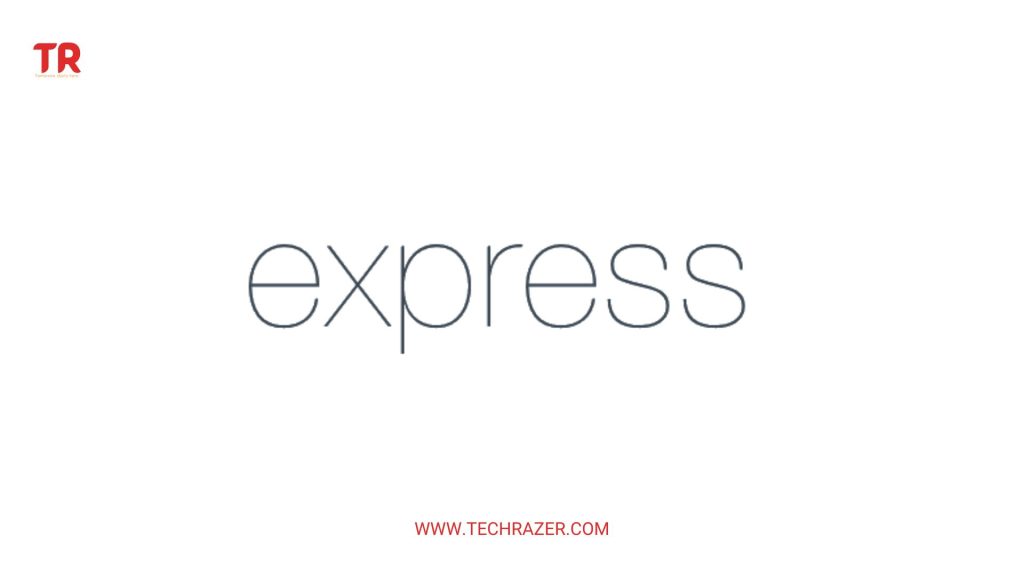
Introduction
ExpressJS, or simply Express, is a minimalist web framework for Node.js, a popular platform for building web applications using JavaScript. It is designed to make the process of building web applications easier by providing a simple set of features that enable developers to create servers, routes, and middleware for handling HTTP requests and responses.
Express was created in 2010 by TJ Holowaychuk and has since become one of the most popular web frameworks for Node.js, with more than 4 million weekly downloads on npm, the Node.js package manager. It is known for its simplicity, flexibility, and performance, and is used by thousands of developers and companies to build a wide variety of web applications, including APIs, single-page applications, and full-stack web applications.
One of the key features of Express is its ability to handle routing, which refers to the process of mapping HTTP requests to specific actions or functions. With Express, developers can define routes for different HTTP methods (e.g. GET, POST, PUT, DELETE) and URLs, and specify the actions or functions that should be executed when a particular route is matched. For example, a route might be defined to handle a GET request to the root URL of a website, or a POST request to a specific URL for creating a new user.
Express also provides a number of middleware functions that can be used to perform tasks such as parsing HTTP request bodies, serving static files, and handling errors. Middleware functions are functions that are executed before the final route handler, and can be used to modify the request and response objects, or perform other tasks such as validation or authentication.
For example, the following code shows how to use the body-parser
middleware to parse the body of a POST request and send a JSON response:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
app.post('/users', (req, res) => {
const user = req.body;
res.json(user);
});
Express also makes it easy to create and use middleware for specific routes or groups of routes. This allows developers to reuse middleware functions and avoid repeating code. For example, the following code shows how to use the authenticationMiddleware
function as middleware for a specific route:
const express = require('express');
const authenticationMiddleware = require('./authenticationMiddleware');
const app = express();
app.post('/users', authenticationMiddleware, (req, res) => {
// code to create a new user
});
In addition to routing and middleware, Express also provides a number of other features that make it a powerful and flexible web framework. These include the ability to set and use templates for rendering HTML pages, support for various HTTP methods and status codes, and integration with other popular Node.js libraries such as Socket.io for real-time communication and Passport for authentication.
Express is also highly extensible, with a large ecosystem of plugins and modules that can be used to add additional functionality to applications. For example, developers can use the express-session
module to add session management to their applications, or the express-validator
module to add request validation.
Popular Use-Cases
ExpressJS is a popular web framework for Node.js that is used to build a wide variety of web applications, including APIs, single-page applications, and full-stack web applications. Some common use cases for Express include:
- Building APIs: Express is commonly used to build APIs that enable different systems or clients to communicate with each other over the web. APIs can be used to expose data or functionality to other systems or developers, or to enable integration between different systems.
To build an API with Express, developers can define routes for different HTTP methods and URLs, and specify the actions or functions that should be executed when a particular route is matched. For example, a route might be defined to handle a GET request to retrieve a list of users, or a POST request to create a new user.
Middleware functions can also be used to perform tasks such as parsing HTTP request bodies, validating input data, or authenticating requests.
For example, the following code shows how to define a route for retrieving a list of users from a database:
const express = require('express');
const app = express();
app.get('/users', (req, res) => {
const users = database.getUsers();
res.json(users);
});
- Building single-page applications: Single-page applications (SPAs) are web applications that load a single HTML page and dynamically update the page as the user interacts with the application. SPAs are popular because they provide a smooth and responsive user experience, and can be built using modern front-end technologies such as React, Angular, or Vue.js.
Express can be used to build the backend of an SPA by providing routes for retrieving data, storing data, and handling other tasks such as authentication or authorization. The backend can then be accessed by the front-end using AJAX requests, enabling the SPA to dynamically update the page without reloading it.
For example, the following code shows how to define a route for creating a new user using a POST request:
const express = require('express');
const app = express();
app.post('/users', (req, res) => {
const user = req.body;
database.createUser(user);
res.json({ message: 'User created successfully' });
});
- Building full-stack web applications: Full-stack web applications are web applications that have both a front-end and a back-end, and handle all aspects of the application from the user interface to the database. Express can be used to build the backend of a full-stack web application, providing routes for handling HTTP requests, interacting with a database, and rendering HTML pages.
To build a full-stack web application with Express, developers can use templates such as EJS or Pug to render HTML pages and pass data from the backend to the front-end. They can also use middleware functions to perform tasks such as parsing HTTP request bodies, serving static files, or handling errors.
For example, the following code shows how to define a route for rendering an HTML page using the EJS template engine:
const express = require('express');
const app = express();
app.set('view engine', 'ejs');
app.get('/', (req, res) => {
res.render('index', { title: 'Welcome to my application' });
});
Benefits of ExpressJS
ExpressJS is a popular web framework for Node.js that is known for its simplicity, flexibility, and performance. Some of the benefits of using Express include:
- Simplicity: One of the main benefits of Express is its simplicity. The framework is designed to be minimalistic, providing only the essential features that are needed to build web applications. This makes it easy for developers to get started with Express and build web applications quickly, without the need to learn a large and complex framework.
- Flexibility: Express is highly flexible and allows developers to customize the way their applications behave. Developers can define their own routes, middleware functions, and templates, and can use various libraries and modules to extend the functionality of their applications. This allows developers to build web applications that meet their specific requirements and needs.
- Performance: ExpressJs is known for its high performance and is able to handle a large number of requests efficiently. The framework is built on top of Node.js, which is known for its fast and scalable runtime, and uses non-blocking I/O to handle requests asynchronously. This enables Express to handle a high volume of requests without becoming bogged down or slowing down.
- Extensibility: ExpressJs has a large ecosystem of plugins and modules that can be used to add additional functionality to applications. These plugins and modules cover a wide range of features, such as session management, authentication, validation, and more, and can be easily integrated into Express applications. This makes it easy for developers to extend the capabilities of their applications and build more powerful and feature-rich web applications.
- Community support: ExpressJs has a large and active community of developers who contribute to the framework and create plugins and modules to extend its functionality. This community support is a valuable resource for developers, as it provides access to a wealth of knowledge and resources, and enables developers to get help and support when they encounter issues or need guidance.
In summary, ExpressJS is a powerful and easy-to-use web framework that is well-suited for building a wide variety of web applications using Node.js. Its simplicity, flexibility, performance, and extensibility make it a popular choice among developers, and its strong community support ensures that developers have access to the resources and support they need to build successful web applications.
Conclusion
ExpressJS is a minimalist web framework for Node.js that is designed to make it easy for developers to build web applications. It is known for its simplicity, flexibility, and performance, and is used by thousands of developers and companies to build a wide variety of web applications, including APIs, single-page applications, and full-stack web applications.
One of the key features of ExpressJS is its ability to handle routing, which enables developers to define routes for different HTTP methods and URLs, and specify the actions or functions that should be executed when a particular route is matched. ExpressJS also provides a number of middleware functions that can be used to perform tasks such as parsing HTTP request bodies, serving static files, and handling errors.
In addition to routing and middleware, ExpressJS also provides a number of other features that make it a powerful and flexible web framework. These include the ability to set and use templates for rendering HTML pages, support for various HTTP methods and status codes, and integration with other popular Node.js libraries.
ExpressJs is highly extensible, with a large ecosystem of plugins and modules that can be used to add additional functionality to applications. It is also supported by a large and active community of developers who contribute to the framework and create plugins and modules to extend its functionality.
Overall, ExpressJs is a lightweight and easy-to-use web framework that is well-suited for building a wide variety of web applications using Node.js. Its simplicity, flexibility, and performance make it a popular choice among developers, and its strong community support ensures that developers have access to the resources and support they need to build successful web applications.