Laravel PHP Framework
Table of Contents
- Introduction: Introduce the Laravel framework and its purpose.
- Benefits of Using Laravel
- Getting Started with Laravel
- Building Blocks: Introduce the building blocks of a Laravel application, such as routes, controllers, views, and models.
- Security: Discuss the security measures that Laravel provides, such as authentication, authorization, and encryption.
- Deployment: Explain how to deploy a Laravel application, such as using a package manager, hosting provider, and database.
- Conclusion
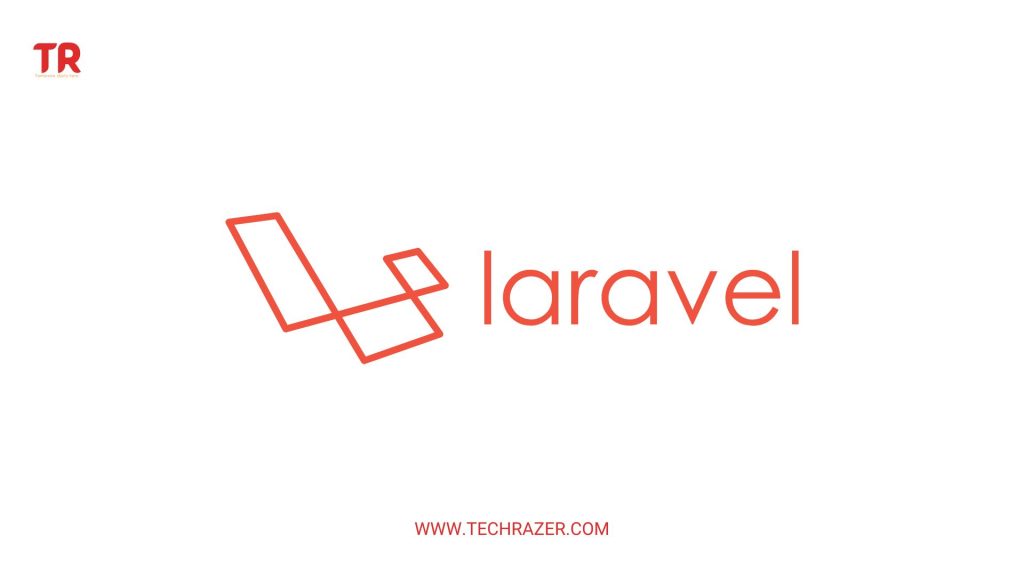
Introduction: Introduce the Laravel framework and its purpose.
Laravel is an open-source web application framework written in PHP. It follows the Model-View-Controller (MVC) architectural pattern and provides an expressive, elegant syntax for web development. It is designed to make the development process easier, more efficient, and more enjoyable.
The main purpose of Laravel is to provide developers with an accessible and efficient toolkit for building modern, feature-rich web applications. It is a full-stack framework that includes an extensive set of features, including routing, authentication, caching, sessions, and more. It also provides a wide range of powerful tools, such as Eloquent ORM and Blade Templating, that allow developers to quickly develop robust applications.
Laravel is designed to make the development process easier and more enjoyable. It is built on top of the Symfony HTTP Foundation component and uses a number of components from the Symfony framework, such as Routing, Request, and Session components. It also provides a variety of useful helper methods and libraries that make common tasks simpler and more efficient.
Laravel also comes with a variety of components that make it easy to create powerful web applications. These components include the Laravel Router, Eloquent ORM, Artisan command-line interface, Blade Template Engine, and more. These components provide developers with an easy way to quickly develop web applications.
In addition to its component-based architecture, Laravel also provides a variety of tools and libraries that make development easier and more enjoyable. These tools include the Laravel Mix tool, which simplifies asset compilation, and the Laravel Valet tool, which allows developers to quickly set up local web servers.
Laravel also provides a variety of services, such as its Forge and Envoyer services, which make deploying web applications easier. It also includes a number of other features, such as the Homestead virtual machine, which provides an isolated development environment, and the Envoy task runner, which makes automating tasks simpler.
Benefits of Using Laravel
Laravel is an open-source, powerful and modern web application framework used for creating web applications. The framework is based on the Model-View-Controller (MVC) architectural pattern. It provides an excellent platform for developing robust, feature-rich websites and applications. It has a wide range of benefits and makes web development easier and faster.
1. Easy to Use and Understand:
Laravel is a web application framework designed to make web development easier and faster. It is a popular choice for many developers because of its intuitive syntax and easy to understand structure. The syntax of Laravel is very straightforward, making it easy for even the most inexperienced developers to understand. The structure of the framework is also designed in a way that makes it easy to modify and extend the code.
2. Built-in Authentication and Authorization:
Laravel offers built-in authentication and authorization features. This allows developers to quickly and easily create secure and robust web applications. It also provides a secure way for users to register and log in to the application. The authentication and authorization features are built in to the framework, so developers do not have to write additional code to make them work.
3. MVC Architecture:
Laravel is based on the Model-View-Controller (MVC) architectural pattern. This pattern makes it easier to separate the logic of the application from the presentation. With MVC, developers can create different models to represent different parts of the application, such as users, posts, comments, etc. The view is used to display the user interface, while the controller handles the logic of the application. This makes it easier for developers to create maintainable and extensible applications.
4. Object-Oriented Libraries:
Laravel comes with a set of object-oriented libraries that make it easier to create robust and secure web applications. The libraries come with features that help developers authenticate users, handle sessions, send emails, and perform other tasks. This makes it easier for developers to create applications that are more secure and reliable.
5. Unit Testing:
Laravel also offers unit testing capabilities. Unit testing is a process in which developers can test their code to ensure that it works as expected. This helps developers find and fix bugs before they become major issues. Unit testing is an important part of development, and Laravel makes it easy to do.
6. Blade Templating Engine:
Laravel also comes with a powerful templating engine called Blade. This templating engine makes it easy for developers to create dynamic and attractive views for their applications. Blade also has a number of built-in features that make it easier to create views that are both secure and performant.
7. Artisan Console:
Laravel also comes with an Artisan console. This console makes it easy for developers to perform tasks such as creating databases, running migrations, and seeding data. It also makes it easier to manage and deploy applications.
8. Database Migrations and Seeding:
Laravel also makes it easy to manage and seed databases. Database migrations are used to create, modify, and delete tables and columns in the database. Database seeding is used to populate the database with test data. This makes it easier for developers to test their applications and ensure that they are working as expected.
9. Automation:
Laravel also offers a number of automation tools. These tools make it easy for developers to automate tasks such as deployment, testing, and database management. This makes it easier for developers to manage their applications and ensure that they are running smoothly.
10. Security:
Laravel also offers a number of security features that make it easy for developers to create secure applications. The framework comes with features such as password hashing, encryption, and authentication. This makes it easier for developers to create applications that are secure and reliable.
Getting Started with Laravel
Getting started with Laravel is a straightforward process and requires minimal setup. To begin, you will need to download and install the appropriate version of the Laravel framework. This can be done by either downloading it directly from the website or by using Composer, a package manager for PHP.
Once you have installed the framework, it is time to create a Laravel project. This can be done by running the artisan command, which is available in the root directory of the Laravel installation. This command creates a ‘Laravel’ directory and automatically creates the necessary files and directories.
The next step is to configure the database. In order to do this, you will need to create a database in your local environment and configure it in the .env file. This file is located in the root directory of the project.
Once the database has been configured, it is time to create the controllers and models. The controllers are responsible for handling the requests from the user and the models represent the data stored in the database. The models and controllers should be placed in the ‘app’ directory of the project.
Once the models and controllers have been created, it is time to write the routes. The routes are responsible for mapping the URL to the appropriate controller and action. The routes should be placed in the ‘routes’ directory of the project.
After the routes have been written, it is time to create the views. The views are responsible for displaying the data to the user. The views should be placed in the ‘resources’ directory of the project.
After the views have been created, the next step is to create the assets. The assets are responsible for providing the necessary CSS and JavaScript files for the project. The assets should be placed in the ‘public’ directory of the project.
Once the assets have been created, it is time to create the migrations. The migrations are responsible for creating and modifying the database tables. The migrations should be placed in the ‘database/migrations’ directory of the project.
Finally, it is time to create the seeds. The seeds are responsible for populating the database with the necessary data. The seeds should be placed in the ‘database/seeds’ directory of the project.
Now that all of the necessary components have been created, it is time to start the server. This can be done by running the artisan serve command. This command will start a web server on the local machine and allow the user to view the project in the browser.
Once the project is running, it is time to begin developing the application. This can be done by writing the necessary code in the controllers and views.
Laravel is a powerful framework that makes it easy to create modern web applications. By following the steps outlined above, you can get started with Laravel quickly and easily.
Building Blocks: Introduce the building blocks of a Laravel application, such as routes, controllers, views, and models.
The Laravel framework is built on a set of components that provide developers with the tools to build applications quickly and efficiently. These components include routes, controllers, views, models, and migrations.
Routes:
Routes are the first building block of a Laravel application. Routes are used to define the URL structure of your application and how a browser should be directed when a particular URL is requested. The routes are stored in a file called routes.php, which is located in the root directory of your project. The routes define the URL patterns and the controller methods that should be called when a request is made.
Controller:
Controllers are the second building block of a Laravel application. Controllers are used to contain the logic that your application needs to execute in response to a request. Controllers are typically stored in a folder called controllers, which is located in the root directory of your project. Controllers are responsible for receiving a request, determining what needs to be done, and then sending a response back to the browser.
Views:
Views are the third building block of a Laravel application. Views are used to create the HTML output that is sent to the browser when a request is made. Views are typically stored in a folder called views, which is located in the root directory of your project. Views are responsible for taking data and transforming it into an HTML page for the user to view.
Models:
Models are the fourth building block of a Laravel application. Models are used to interact with a database and provide an interface to the data stored within it. Models are typically stored in a folder called models, which is located in the root directory of your project. Models are responsible for retrieving and manipulating data from the database.
Migrations:
Migrations are the fifth building block of a Laravel application. Migrations are used to define the structure of a database. Migrations are typically stored in a folder called migrations, which is located in the root directory of your project. Migrations are responsible for creating and modifying tables, columns, and other database objects.
These five building blocks are the basic foundation for any Laravel application. Each of these components plays an integral role in allowing developers to create applications quickly and easily. By using these components, developers can create robust applications that are secure, modern, and user-friendly.
Security: Discuss the security measures that Laravel provides, such as authentication, authorization, and encryption.
Security is a top priority for any software developer, and Laravel is no exception. Laravel is a popular open source web application framework which has been designed to make development easier and more secure. It provides a range of features which help to protect applications from malicious attacks. In this article, we will discuss the security measures that Laravel provides, such as authentication, authorization, and encryption.
Authentication
Authentication is the process of verifying the identity of a user before allowing them access to a system or application. This is an essential part of keeping an application secure. Laravel provides a built-in authentication system which is easy to set up and use. It uses the standard PHP password hashing algorithm, bcrypt, to ensure that passwords are secure. It also provides support for social media authentication, allowing users to log in with their existing accounts from major providers, such as Facebook and Google.
Authorization
Authorization is the process of determining which users have access to which parts of an application. Laravel provides an authorization system which allows developers to define who has access to which parts of the application. This system is based on roles and permissions which can be configured to give different levels of access to different users. This ensures that the application is secure and only users with the correct permissions can access the parts of the application they need to.
Encryption
Encryption is the process of transforming data into a form which is unreadable and secure. This ensures that the data is kept safe from malicious actors. Laravel provides support for a range of encryption algorithms, including AES-256, which is one of the most secure encryption algorithms available. It also provides support for storing encrypted data in a database, ensuring that the data is kept secure even if the database is compromised.
Deployment: Explain how to deploy a Laravel application, such as using a package manager, hosting provider, and database.
Deploying a Laravel application can be a daunting task for the inexperienced developer. However, with the right tools and knowledge, it can be a straightforward process. This tutorial will guide you through the process of deploying your Laravel application.
First, you will need to choose a package manager. Package managers are used to manage the software dependencies required for your application. Laravel supports a number of package managers, such as Composer, NPM, and Bower. Each of these has their own advantages and disadvantages, so make sure to choose the one that is best suited to your needs.
Once you have chosen a package manager, you will need to set up the hosting environment for your application. There are a number of hosting providers available, such as Amazon Web Services, DigitalOcean, and Linode. You will need to choose a provider that meets the requirements of your application.
Once your hosting environment is set up, you will need to install the necessary software packages. This includes the web server, database, and other components. For the web server, you will need to install Apache or Nginx. For the database, you will need to install MySQL, PostgreSQL, or SQLite.
Once the software packages have been installed, you can begin the process of deploying your application. To do this, you will first need to upload your application files to your server. This can be done using FTP or SFTP. Once the files have been uploaded, you will need to install the necessary dependencies using your package manager.
Once the dependencies have been installed, you will need to configure your application for the server environment. This includes setting up the web server configuration, database connection, and other environment variables. This can be done using the Laravel artisan command.
Once your application is configured, you will need to create a database and perform any necessary migrations. This can be done using the Laravel artisan command. Once the database has been created, you will need to configure your application to connect to it.
Once your application is configured and the database is set up, you are ready to deploy your application. This is done by running the Laravel artisan command. If everything is set up correctly, your application should now be accessible to the public.
Deploying a Laravel application can be a challenging task for the inexperienced developer. However, with the right tools and knowledge, it can be a straightforward process. This tutorial has provided you with the necessary steps to deploy your application. Good luck, and happy coding!
Conclusion
In conclusion, Laravel is an incredibly powerful open-source PHP web framework for developing web applications. It is incredibly scalable, flexible, robust, and secure, making it ideal for creating applications that are safe and secure. Additionally, it provides a wide range of tools and features to make development faster and easier. Getting started with Laravel is easy, and developers can quickly create and deploy web applications using the tools it provides.