Getting Started with Python Programming
Table of Contents
- Introduction
- Benefits of Python
- Python Syntax
- Python Structure
- Python Libraries
- Applications Of Python
- Conclusion
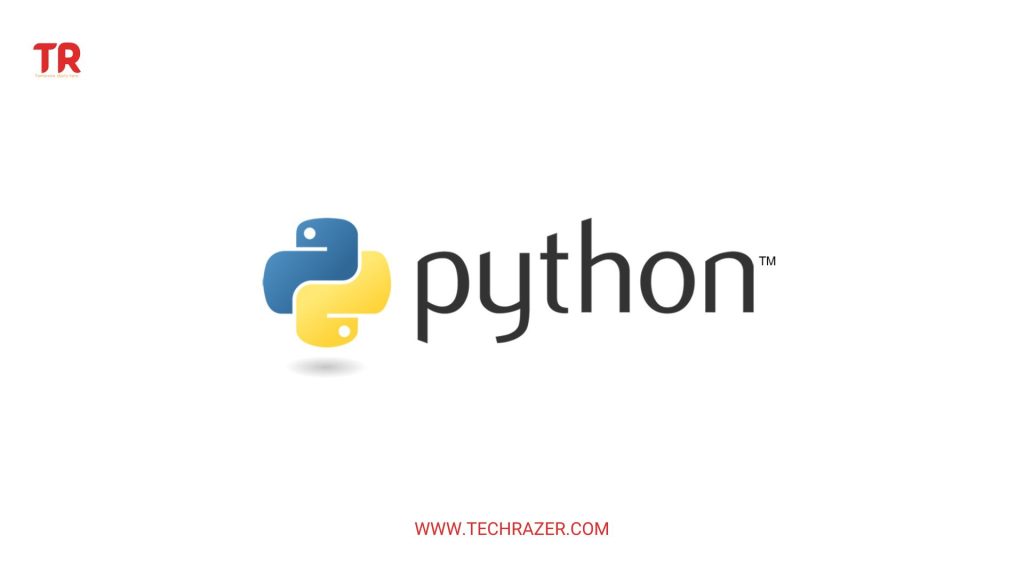
Introduction
Python is a high-level, object-oriented programming language with a focus on code readability and ease of use. It is used by many software developers and data scientists to develop a wide range of applications, from web applications to data science projects. Python was originally developed in the late 1980s by Dutch programmer Guido van Rossum and was first released in 1991. It was designed to be a simple, powerful, and easy-to-learn language for programming.
Python has become a popular language for many reasons. One of the main reasons is that it is an open-source language, meaning that anyone can access the source code and modify it, allowing it to be used for a variety of different applications. Additionally, Python is a versatile language, allowing it to be used for a wide range of tasks, from web development to machine learning. Finally, Python has a large and active community of developers who are continuously contributing to the language, making it easy to find help and resources when needed.
The history of Python dates back to the late 1980s, when Dutch programmer Guido van Rossum began working on what would become the Python programming language. Van Rossum wanted to create a language that was easy to use and understand, and he began designing the language in the early 1990s. The first version of Python was released in 1991 and was quickly adopted by many developers and organizations.
The purpose of Python is to provide a powerful, easy-to-use language for programming. Python was designed to be an accessible language, allowing developers to quickly and easily create software without having to learn a complex language. Python is also an interpretive language, meaning that it can interpret code as it is being written, making it easier to debug and test. Finally, Python is a multi-paradigm language, allowing developers to use a variety of programming styles, such as procedural, object-oriented, and functional programming.
Python has become a popular programming language for many reasons. One of the main reasons is its readability and ease of use. Python is designed to be a simple language, allowing developers to quickly and easily create software without having to learn a complex language. Additionally, Python is an interpretive language, making it easier to debug and test. Finally, Python has a large and active community of developers who are continuously contributing to the language, making it easy to find help and resources when needed.
The popularity of Python has grown steadily over the years, with the language being used for a wide range of applications, from web development to data science. In recent years, Python has become especially popular for data science applications, with many organizations using it for machine learning and data analysis. This popularity is due to the ease of use of the language, as well as its powerful libraries, such as NumPy, Pandas, and SciPy, which provide powerful tools for data analysis.
In order to use Python, the first step is to install the Python interpreter. This can be done on Windows, Mac, and Linux systems, and is typically done using an installation wizard. After the interpreter is installed, Python code can be written in a text editor such as Notepad or TextWrangler. After the code is written, it can be run using the Python interpreter. This will execute the code, and any output will be displayed in the console window.
The next step is to learn the basics of Python. This includes understanding the syntax, data types, and basic programming concepts. There are many tutorials and books available to learn the basics of Python, and the official Python website also provides an excellent tutorials.
Once the basics of Python are understood, it is important to learn how to use the language to create applications. This includes understanding how to use the standard library, as well as how to use third-party packages and libraries. Additionally, it is important to learn how to debug and test applications, as well as how to deploy them.
It is important to stay up to date with the Python language. This includes understanding the latest features and updates, as well as participating in the Python community. By staying up to date, developers can ensure that their applications are taking advantage of the latest features and technologies.
Benefits of Python
Python is a general-purpose, high-level programming language used for the development of applications, websites, software, and games. Python is an interpreted language, meaning it does not need to be compiled prior to running. This makes Python programming significantly easier than other languages such as C++ and Java. Python is also an object-oriented language, meaning it is organized around objects rather than actions and data rather than logic. Python is also cross-platform, meaning it can be run on multiple operating systems such as Windows, Mac OS X, and Linux.
- Easy to Learn and Use:
Python is an easy to learn, powerful language. It uses simple, easy-to-understand syntax and has a large library of standard functions and modules. This makes it easy for beginners to get up and running with the language quickly. Python also has extensive online documentation and resources available, so learning how to use the language is easy. - Increased Productivity:
Python enables developers to write programs in fewer lines of code than other languages, making it more efficient and productive. This means developers can focus on the core functionality of their applications, rather than writing code for mundane tasks. Additionally, the large standard library of functions and modules means developers can quickly find the code they need, so they don’t have to spend time writing it from scratch. - Easy to Maintain:
Python is an interpreted language, so it does not need to be compiled prior to running. This makes it easy to maintain and modify existing code as the application evolves. Additionally, Python’s simple syntax and structure make it easy for developers to quickly understand existing code and make changes as needed. - Open Source:
Python is an open source language, meaning it is free to use and modify. This makes it an ideal choice for both individuals and businesses, as there are no upfront costs associated with using the language. Additionally, open source languages are constantly being improved and updated by a large community of developers, so developers can always be sure they are using the most up to date version of the language. - Increased Performance:
Python is an interpreted language, so it can be run on multiple operating systems. This makes it easy to deploy applications on multiple platforms. Additionally, Python’s dynamic typing system and garbage collection make it faster and more efficient than other languages. - Robust Libraries:
Python has a large standard library of functions and modules, making it easy for developers to find the code they need. This means developers don’t have to spend time writing code from scratch, which saves time and increases productivity. Additionally, Python has a large number of third-party libraries, so developers can easily find the code they need for a particular task. - Scalability:
Python is an interpreted language, so it can be used for applications of all sizes. This makes it ideal for both small and large applications, as it can scale easily as needed. Additionally, Python’s modular structure makes it easy to add new features or functions as needed.
Python Syntax
Python is a very simple language and uses a very intuitive syntax. The syntax is based on indentation, which is used to mark blocks of code. This means that Python code is easy to read and understand, and makes it easier for beginners to learn the language.
Python uses a variety of data types, such as integers, floats, strings, and lists. There are also a variety of built-in functions and modules, which allow you to perform a wide range of tasks. Python also supports object-oriented programming, which allows you to create complex programs with a modular approach.
Python also has a large number of libraries, which are packages of code that allow you to perform a variety of tasks. These libraries are often called ?modules?, and allow you to access a range of functions and classes.
Python Structure
Python programs are structured in a way that helps make them easier to read and debug. The structure of a Python program consists of three parts:
- The main file: This is the main file of the program, which contains the main code. This file is usually named ‘main.py’, and is the starting point of the program.
- The modules: These are the additional files that contain code that can be imported into the main file. Modules are often used to separate code into different parts or to provide reusable functions.
- The classes: Classes are used to group together related functions, and to create objects that can be used throughout the program.
The main file of a Python program is usually very simple and consists of a series of import statements, functions, and classes. It is usually the first file that is executed when the program runs.
The modules are imported into the main file, and are usually used to separate code into different parts. This makes the code easier to read and debug. Modules are imported using the ‘import’ statement.
The classes are used to group together related functions, and to create objects that can be used throughout the program. Classes are created using the ‘class’ statement.
Python also has a large number of built-in functions, which are used to perform a variety of tasks. These functions are used to manipulate data, and can be used to create complex programs.
Python Libraries
Python libraries are a collection of pre-written code that can be used by developers and programmers to simplify the development process. Python libraries are important because they provide a set of powerful tools that can be used to quickly and efficiently develop powerful applications.
Python is an interpreted programming language, which means it is interpreted and executed at runtime. This makes it an excellent language for rapid application development. However, Python code can become complex and time consuming to write. Python libraries provide a way to reduce development time and make coding easier.
Python libraries are collections of modules, classes, functions, and other code objects that are written in Python and can be imported into a Python program to be used. Libraries are often used to shorten development time and provide common functionality which would otherwise require a lot of custom programming. For example, the csv library can be used to easily read and write data from a csv file without having to write custom code.
Python libraries are also used to extend the functionality of Python. For example, the NumPy library provides powerful tools for working with numerical data. The SciPy library provides a variety of scientific computing tools.
Python libraries are also important because they provide a way to reuse code. If a developer has written a library that is useful, they can share it with other developers who can incorporate it into their own projects. This means that developers don’t have to start from scratch each time they need to write a piece of code.
Python libraries are also important because they provide a way to maintain code quality. Libraries are often tested and debugged before they are released, which can help ensure that they are free of bugs and are optimized for performance. This can save developers time and money in the long run.
Python libraries are also important because they provide a way to ensure that code is portable and cross-platform compatible. Libraries written in Python can often be used on different operating systems with minimal changes. This means that developers can write code once and have it run on multiple platforms without having to rewrite it.
Applications Of Python
Python is a powerful and popular programming language. It is used for a wide range of applications, such as web development, data science, machine learning, and artificial intelligence (AI). Python is a versatile language and has been used to create a variety of applications, from simple scripts to large-scale enterprise applications. In this article, we will discuss the various applications of Python and their implications in the current tech industry.
- Web Development
Python is a popular language for web development, due to its ease of use and flexibility. It is used for creating dynamic web pages and web applications. Python is used for server-side scripting, which is the code that runs on the server to generate the web pages. Python is also used for creating web services, which are applications that allow users to interact with the server from their web browser. Python offers a range of libraries and frameworks for web development, such as Django, Flask, and Pyramid, that make it easier to create web applications.
- Data Science
Data science is the process of extracting insights from structured and unstructured data. Python is a popular language for data science due to its wide range of libraries and frameworks for data analysis. Python is used for data wrangling, which is the process of cleaning and formatting data for analysis. It is also used for data visualization, which is the process of creating graphs and charts to visualize data. Python is used for machine learning, which is the process of training a computer to make predictions and decisions based on data. Python is also used for natural language processing, which is the process of understanding and analyzing text.
- Machine Learning
Machine learning is a subfield of artificial intelligence that enables computers to learn from data without being explicitly programmed. Python is a popular language for machine learning due to its wide range of libraries and frameworks. Python is used for supervised learning, which is the process of training a machine learning model using labeled data. It is also used for unsupervised learning, which is the process of training a machine learning model using unlabeled data. Python is used for deep learning, which is a type of machine learning that uses neural networks to learn from data.
- Artificial Intelligence
Artificial Intelligence (AI) is the process of enabling machines to think and act like humans. Python is a popular language for AI due to its wide range of libraries and frameworks. Python is used for computer vision, which is the process of enabling machines to recognize objects from images or videos. It is also used for natural language processing, which is the process of understanding and analyzing text. Python is used for robotics, which is the process of creating autonomous robots that can interact with the environment.
Python has become an essential tool for a wide range of applications, from web development and data science to machine learning and AI. Python is a versatile language and has been used to create a variety of applications, from simple scripts to large-scale enterprise applications. It is a popular language due to its wide range of libraries and frameworks, which makes it easier to create powerful applications. Python is a powerful language and its applications are only going to grow in the future.
Conclusion
In conclusion, Python is a powerful, versatile programming language that offers many benefits. It is easy to learn and use, increasing developer productivity. It is open source, so there are no upfront costs associated with using the language. It also has a large standard library of functions and modules, making it easy to find the code you need. Additionally, Python is an interpreted language, so it can be used for applications of all sizes and can be run on multiple operating systems. All of these benefits make Python an ideal choice for both individual developers and businesses.